Grid layouts
ANU websites are designed based on the Grid system of Bootstrap version 5. The viewport is 1200px wide, and we are using the grid system to divide the webpage into 12 columns with equal widths.
If you are familiar with our Grid layouts or have experience with Bootstrap Grid layouts. You can use the image here as a quick reference, it demonstrates some common combinations of column items.
Each row in the diagram represents a particular layout structure. For example, the 3rd row represents a 2-column layout. When the viewport is wider than the sm
breakpoint, the left column will take up 8/12 (which is 2/3) of the viewport while the right one takes up the rest 4/12 (which is 1/3) of the viewport.
Read below for an in-depth explanation of how the grid system works.
Overview
This layout is fully responsive, we can control the webpage layout by using the following classes. The classes are named using the format col-{breakpoint*}-{column width}
, such as .col-md-6
or .col-12
. *Breakpoint is an optional field.
Breakpoints are also known as responsive breakpoints. Breakpoints are based on different screen sizes, such as mobiles, laptops, etc. Applying breakpoints will let the web content adapt to a certain layout design in order to provide a better user experience.
Where breakpoint is one of:
Breakpoint | Description | Dimension |
---|---|---|
xs | Extra small | <576px |
sm | Small | ≥576px |
md | Medium | ≥768px |
lg | Large | ≥992px |
xl | Extra large | ≥1200px |
xxl | Extra extra large | ≥1400px |
Column width can be any number from 1 to 12, which stands for the number of columns that the div spans.
You don't need to define all grid layout styles for each breakpoint that we included in the table. In our practice, the 3 most common breakpoints are sm, md, lg
, and the most common column widths are: 3, 4, 6, 8, 9, 12
.
Gutter
Our grid system has default gutter between each column. In case you want to increase or decrease the gutter size, you can use the following classes to achieve.
g-{size}
Where size can be one of:
0
: set the gutter size to 0rem1
: set the gutter size to 0.25rem2
: set the gutter size to 0.5rem3
: set the gutter size to 1rem4
: set the gutter size to 1.5rem5
: set the gutter size to 3rem
See below as an example:
<div class="container">
<div class="row py-3">
<div class="col-6"><div class="p-3">col-6</div></div>
<div class="col-6"><div class="p-3">col-6</div></div>
</div>
<div class="row py-3 g-0">
<div class="col-6"><div class="p-3">col-6</div></div>
<div class="col-6"><div class="p-3">col-6</div></div>
</div>
</div>
In this example, we can see there was a default gutter between columns. It indicates the default gutter is not zero. If you want to make two columns adjacent to each other, you may want to add g-0
as a class.
Walkthrough examples
Below are a few examples to help you understand the grid class better:
<div class="col-sm-12 col-md-6 col-lg-4">
- Screen size ≥ 992px: the container will take 4 out of 12 columns
- 992px > Screen size ≥ 768px: the container will take 6 out of 12 columns
- 768px > Screen size: the container will take 12 out of 12 columns
<div class="col-12 col-lg-6">
- Screen size ≥ 992px: the container will take 6 out of 12 columns
- 992px > Screen size: the container will take 12 out of 12 columns
<div class="col-12 col-md-6 col-xl-4">
- Screen size ≥ 1200px: the container will take 4 out of 12 columns
- 1200px > Screen size ≥ 768px: the container will take 6 out of 12 columns
- 768px > Screen size: the container will take 12 out of 12 columns
Please refer to the Use cases tab to understand the application of these classes and the overall code structure better.
Use cases
Here we will give a few commonly used layout structures and its corresponding code structure. This will help you to understand how to use the cheatsheet above to layout your page content better.
Content in full width
Use the basic structure container - row - col and put all your content inside of the col
container, and it will take 100% width of this parent container (it's row in this case).
<div class="container">
<div class="row">
<div class="col bg-tint">
<p>Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum.</p>
</div>
</div>
</div>
Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum.
2/3 and 1/3 layout (responsive)
As mentioned in previous sections, the viewport is divided into 12 equal-width columns. So, when we want an element to take two-thirds of the screen, we can do some simple calculation: 12 columns * 2/3 = 8
which means the element will take 8 columns. And the container on the right will take 4 columns.
Also, we need to consider the responsive layout. On smaller screen sizes, if we keep this 2/3 and 1/3 structure, it will make our webpage less accessible. In case of lengthy text contents, it's recommended to make it full width on sm
screen sizes.
<div class="container">
<div class="row equal">
<div class="col-sm-12 col-md-8 bg-tint">
<p>When your screen size is smaller than 768px, this 2/3 container will be transformed to a full width column.</p>
</div>
<div class="col-sm-12 col-md-4">
<div class="box-header-tint large">Box header</div>
<div class="box-bdr-gold">When your screen size is smaller than 768px, this 1/3 container will be transformed to a full width column.
</div>
</div>
</div>
</div>
When your screen size is smaller than 768px, this 2/3 container will be transformed to a full-width column.
3 equal-width columns (responsive)
Like the example above, we are also applying the responsive classes here. As we want each column to take up 1/3 of the viewport on large screen size devices, and expand to the full width of the viewport when the screen size is smaller than 576 pixels.
<div class="container">
<div class="row">
<div class="col-sm-4 bg-tint">
<h4>col-sm-4</h4>
<p>Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum
Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum.</p>
</div>
<div class="col-sm-4 bg-tint">
<h4>col-sm-4</h4>
<p>Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum
Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum.</p>
</div>
<div class="col-sm-4 bg-tint">
<h4>col-sm-4</h4>
<p>Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum
Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum.</p>
</div>
</div>
</div>
col-sm-4
Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum.
col-sm-4
Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum.
col-sm-4
Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum.
Style usage
1. Grid with text overlay
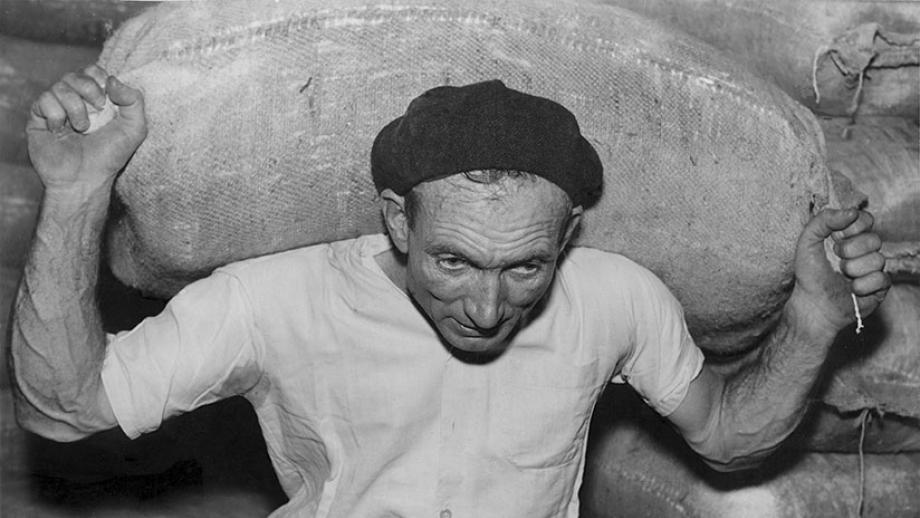
Noel Butlin Archives Centre
The Noel Butlin Archives Centre (NBAC) collects business and labour records from Australian companies, trade unions, industry bodies and professional organisations. We are a national organisation with material from all states and territories. The NBAC holds ...
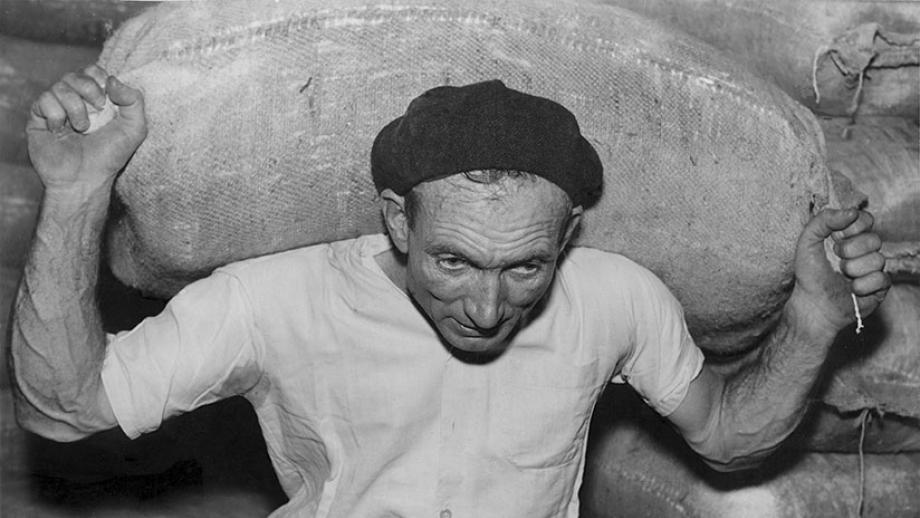
Noel Butlin Archives Centre
The Noel Butlin Archives Centre (NBAC) collects business and labour records from Australian companies, trade unions, industry bodies and professional organisations. We are a national organisation with material from all states and territories. The NBAC holds ..
2. Event grid with date overlay
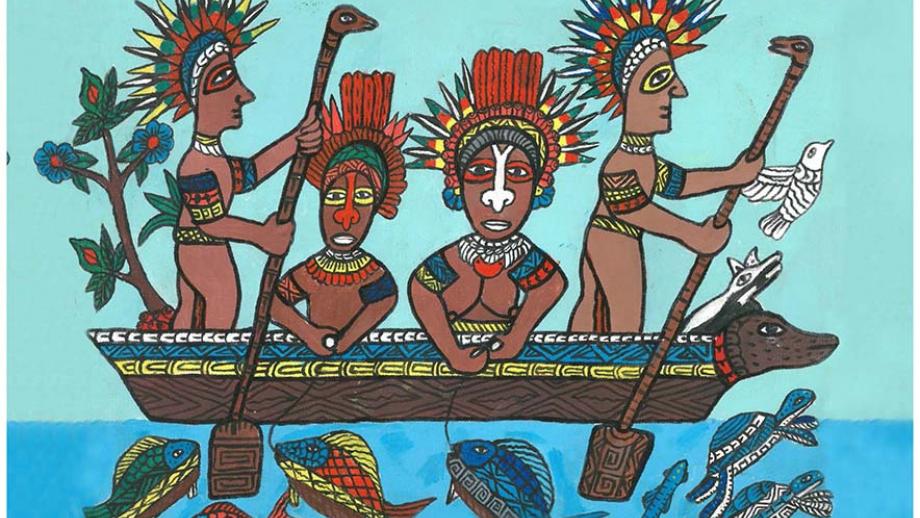
Lorem ipsum dolor sit amet
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
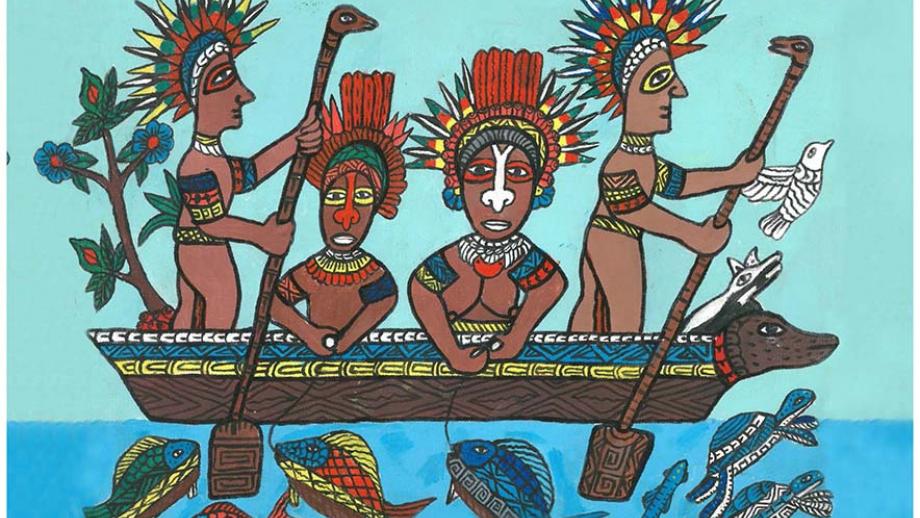
Lorem ipsum dolor sit amet
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
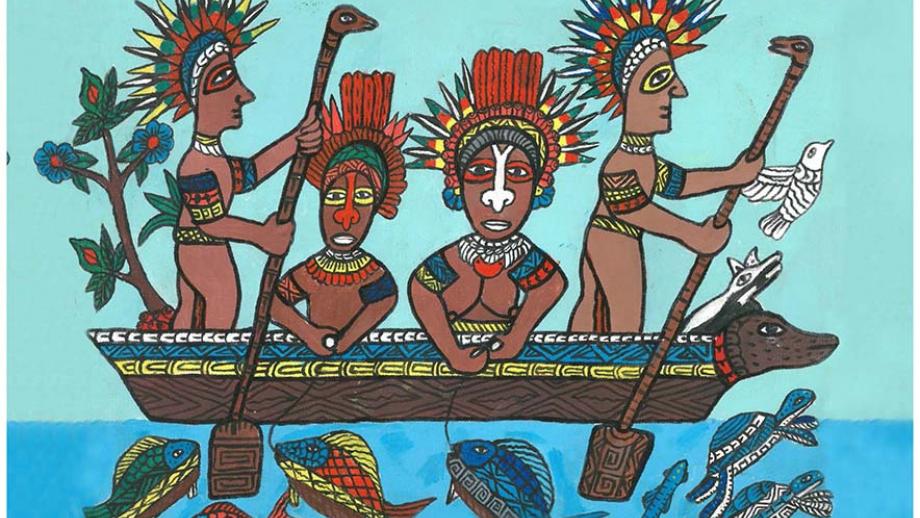
Lorem ipsum dolor sit amet
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
3. News grid with view more button
0. Quick view
If you are already familiar with our Grid layouts or have experience with Bootstrap Grid layout. You can use the example here as a quick reference.
The viewport is divided into 12 columns with equal width. Here is a basic structure of the code:
<div class="container">
<div class="row">
<div class="col"> Your content </div>
<div class="col"> Your content </div>
</div>
</div>
Each row in the diagram represents a particular layout in your webpage. For example, the third row represents a 2-column layout. When the viewport is wider than the sm
breakpoint, the left column will take up 2/3 of the viewport while the right one take up the rest 1/3.
1. Introduction
ANU websites are designed based on the Grid system of Bootstrap version 5. The main layout is 1240 (to be adjusted). And we are using the grid system to divide the webpage into 12 columns.
After you have the standard framework in place, we are using a series of containers, rows, and columns to layout and align content. The layout is fully responsive. Below is an example and an in-depth explanation for how the grid system comes together.
2. How it works
We can see that every col
(column) are contained in a row
, and a row
is inside of a container
. The class name for columns are not limited to col
. It can be extended as a full format like col-**-#
. **
refers to the breakpoints. We have xs
, sm
, md
, lg
, xl
and xxl
from extra small to extra extra large. And the #
can be any number from 1 to 12 which stands for the number of columns (12 in total) that the div
spans.
Breakpoints are based on min-width
media queries, meaning they affect that breakpoint and all those above it. (eg .col-sm-4
applies to sm
, md
, lg
, xl
, and xxl
). This means you can control container and column sizing and behavior by each breakpoint.
In our practice, the three most common columns are sm
(small, the size for phones), md
(medium, the size for tablets), and lg
(large, the size for computers). For example, in the third row of the image, we have col-sm-8
and col-sm-4
. By default, if a smaller breakpoint is not defined (xs
in this example), it would span the whole width. So, when the viewport's width is less than 576 pixels (defined by breakpoints), each of the divs will take up a whole row. And when it is greater than 576 pixels, the two columns will be in the same row, and the one with col-sm-8
with take up 8/12 of the width and the one with col-sm-4
will take up 4/12 of the width.
3. Example layouts
Here we will give 3 examples for the common used layouts. Look into the example, it will help you understand how to use the cheatsheet above to structure your page accordingly.
Content in full width
Use the basic structure container - row - col and put all your content inside of the col tag, and it will automatically occupy the whole width of its contaienr.
Here's the code:
<div class="container">
<div class="row">
<div class="col bg-tint">
<p>Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum.</p>
</div>
</div>
</div>
Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum.
2/3 content and 1/3 box on the right (responsive)
As mentioned above, the whole width of the viewport is divided into 12 equal-width columns. So, when we want an elements to take two thirds of the screen, we can do some simple calculation: 12 columns * 2/3 = 8
which means the element will take 8 columns. And the box on the right will have 4 columns.
Also, we need to consider about the responsive layout. When the screen size goes smaller, if we squeeze the element, it may look ugly. In this case, on the third row of our cheatsheet, we have col-sm-8
. It means only when the screen is wider than "small" which is 576 pixels (medium is 769 pixels), the elements will occupy 8 columns of 12. Or it will take up the whole width.
Here's the code:
<div class="container">
<div class="row">
<div class="col-sm-8 bg-tint">
<p>Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum
Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum.</p>
</div>
<div class="col-sm-4">
<div class="box-header-tint large">Box header</div>
<div class="box-bdr-gold">Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum
</div>
</div>
</div>
</div>
Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum.
3 equal width columns in one row (responsive)
Like the above example, we are also applying the responsive classes here. As we want each column to take up 1/3 of the screen size, there will be 4 columns for each. And the column will go full width when the screen width is smaller than 576 pixels.
Here's the code:
<div class="container">
<div class="row">
<div class="col-sm-4 bg-tint">
<h4>col-sm-4</h4>
<p>Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum
Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum.</p>
</div>
<div class="col-sm-4 bg-tint">
<h4>col-sm-4</h4>
<p>Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum
Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum.</p>
</div>
<div class="col-sm-4 bg-tint">
<h4>col-sm-4</h4>
<p>Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum
Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum.</p>
</div>
</div>
</div>
col-sm-4
Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum.
col-sm-4
Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum.
col-sm-4
Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum Lorem Ipsum.